In the object-oriented programming world, polymorphism is an interesting mechanism that allows methods of a class to take multiple forms. While compile-time polymorphism largely deals with method overloading, runtime polymorphism enhances the simplicity of inheritance, a pillar of the object-oriented programming language, by method overriding.
In Java, a child class has the flexibility to override the parent’s behavior without impacting the parent class.
So, What is Runtime Polymorphism?
It is a technique that allows the JVM to invoke an overridden child method dynamically using the parent reference during runtime. Hence, this is known as dynamic polymorphism.
Since the methods are dynamically resolved, this concept is also known as Dynamic Method Dispatch.
How Does it Work?
A child class overrides a method of the parent class. The overridden method can be invoked using a parent class reference. This is known as upcasting. The compiler will not know as to which method to be called as the method is present in both the parent and the child classes. It is resolved during runtime by the JVM based on the object the parent is referring to.
Runtime polymorphism can be achieved for single and multilevel hierarchy classes. However, if the child classes do not override the method of the parent class, then the parent class method is called during execution.
Let’s simplify it with an example.
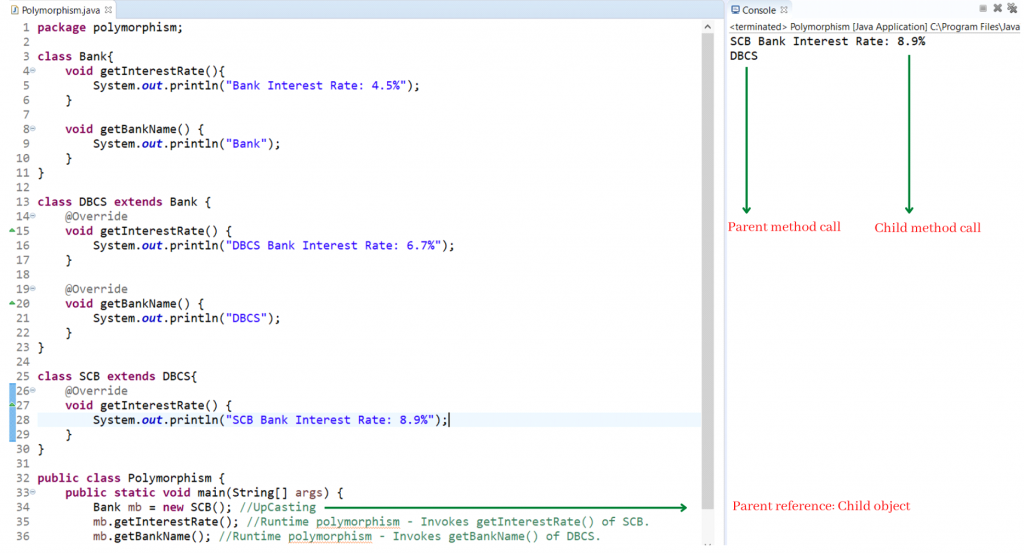
Class Bank contains a method getInterestRate() that displays the interest rate for that bank.
Class DBCS extends Bank, and SCB extends DBCS. Both the DBCS and the SCB banks have overridden the method getInterestRate() from the parent class.
Let’s create an object of class SCB using the Bank reference.
When the getInterestRate() method is called, the JVM invokes the SCB method call during runtime.
Similarly, the JVM calls the method getBankName() from DBCS class as this method is not overridden in the SCB class.
Limitations of Runtime Polymorphism
Although Dynamic polymorphism is useful in many scenarios, there are a few limitations too:
- Runtime polymorphism works only for the methods of a class and not for the variables
- It can be achieved only for the overridden methods